Illustrated Guide to getting started with Golang
Go is a great language by many accounts. When I first got started with Go, I didn't have a lot of experience with programming languages that required a specific workspace or a lot of setup.
Go requires you to set 2 or 3 variables, add stuff to your $PATH
(what?) and also expects you to put your files in a certain directory structure. This can be a bit offputting when you're used to just doing whatever you want.
Full disclosure, this is a less accurate and less informative version of How to Write Go Code from the official website.
Usually with PHP you throw files into a place and the server spits it back out all processed, with ruby and python you run stuff and they both were installed with the Linux I had installed at the time. Node.js was so fresh it had to be installed a little more manually, but it worked relatively easily too in the pattern:
node server.js
and my code ran.
With Go however, we have to decide where we want our projects to live. In order to not get my brain confused excessively, I try to have a directory that's called projects
on all my machines in which I have a directory called go
.
One of the reasons Go is one of my favourite languages, is that the mascot literally looks like it's either vision impaired or currently abusing a substance. Don't forget to create your own go avatar at gopherize.me
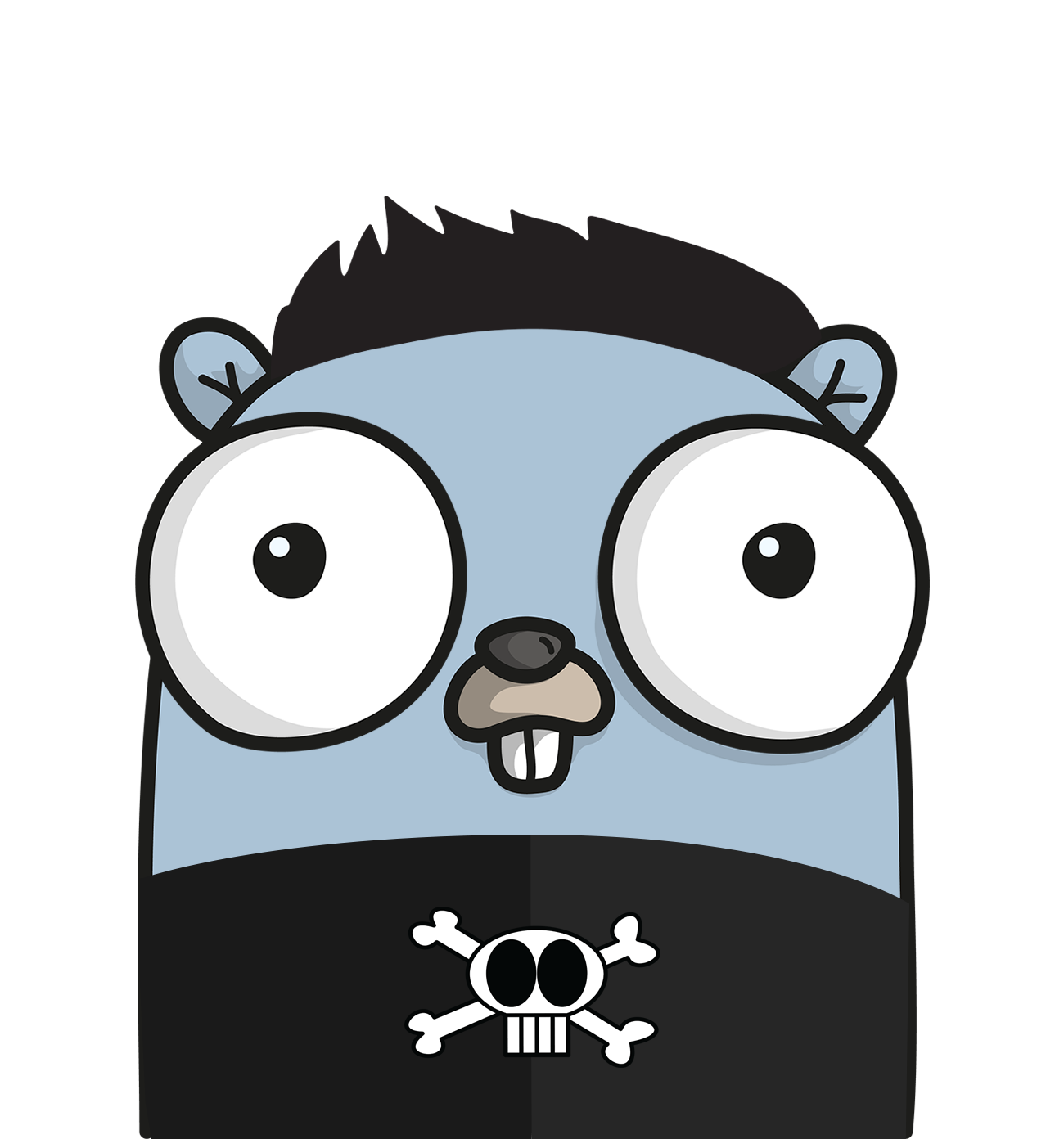
Setting The GOPATH
Go needs to know where your "workspace" is on your computer. This can be a bit cringy for people who're used to having a specific place for all their projects. It kind of forces you to keep all your Golang projects in one place.If you imagine it like a room, you'll need one table (directory) for all of your Go projects.
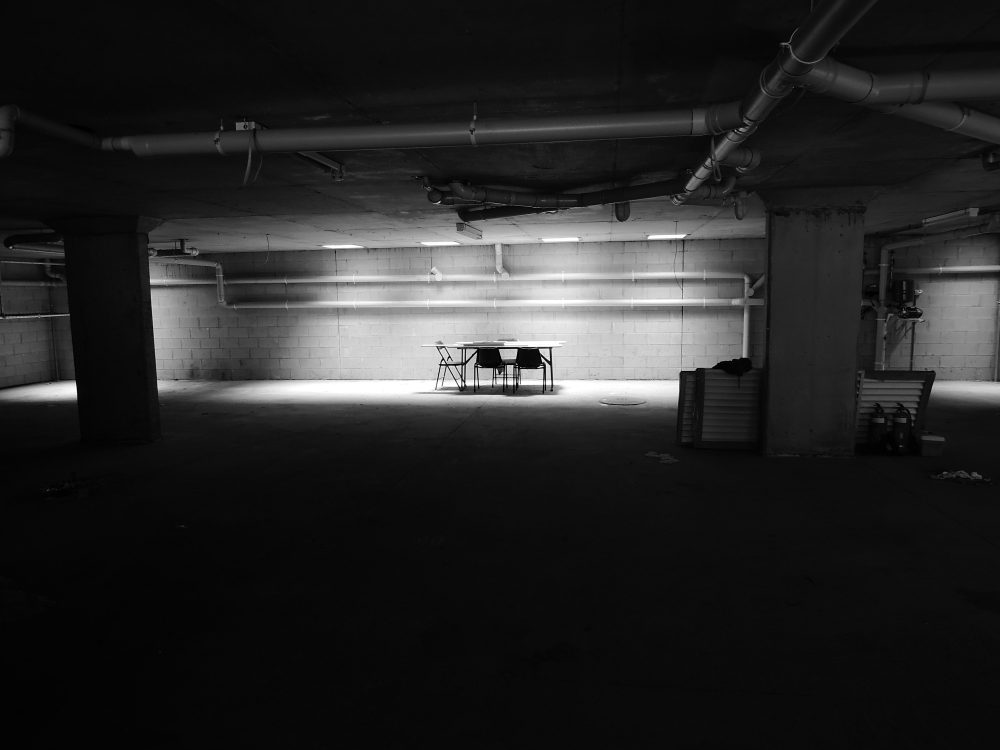
So if you work with code routinely or occasionally, you'll probably have a projects
folder. Mine is at /home/jonathan/projects
for the most part, so I now have one more in that that is called go
and I need to tell my environment about that. Your terminal is probably either bash (on Linux and MacOS) or zsh. If you use something else or Windows, check out the official documentation on setting the GOPATH.
Inside the go
directory
What's inside the Go directory? Mainly, three other directories:
bin
pkg
src
(this is the fun one!)
The src
directory is where you work on your projects, so if you think back to your projects directory we're now here:
projects
-> go
-> src
and here we create our go projects like hello-world
. Let's imagine it like a factory.
The src
is the source code and where the work gets done inside your factory.
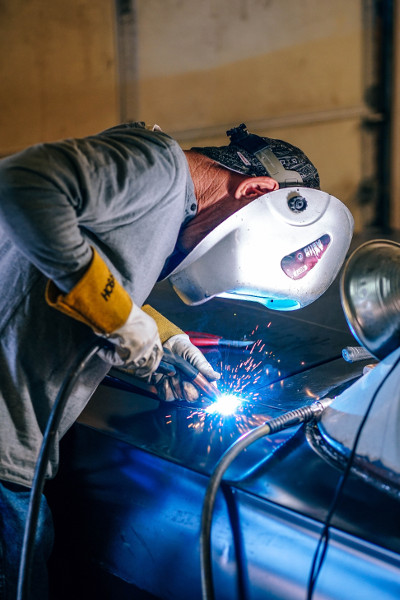
The bin
directory is where the finished products are:
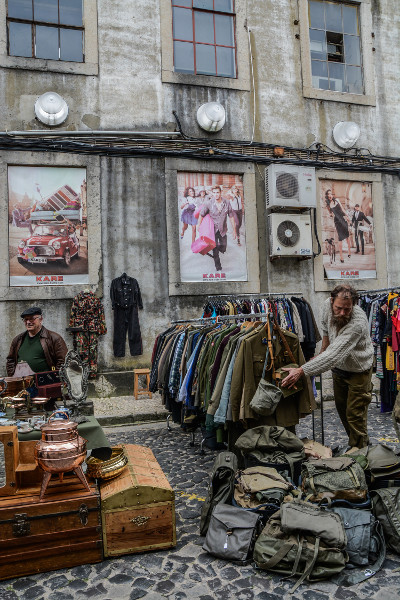
The finished products are your binaries or runnable programs. Since go needs to compile your code in order to execute it, it will place the finished results (and those of other dependencies you install) there.
Hello World in Go
Let's go ahead and create our first program in Go:
Reminder, we're right now in /home/jonathan/projects/go/src/hello-world/
and create a file called hello.go
.
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
- we declare the package (name of our program), for most of your tutorial and test programs you can leave that at
main
import "fmt"
means we want to import the part of the standard library that lets us print stuff to the terminal, likeputs
in ruby orconsole.log()
in JavaScript- the
main()
function, the part of your program that gets run by default. This exists for every program in Go that needs to do something when it is started. Println("Hello World!")
will output to the terminal.fmt
we imported and it has a function calledPrintln
which Prints a line.
You can go ahead and run your program with go run hello.go
now and it should say:
Hello World!
Variables in Go
If we want to have a dynamic Hello World in Go, we can add a variable to the mix:
var something string
something = "World"
fmt.Printf("Hello %s!\n", something)
Note that we switched the Println
to Printf
and the \n
now manually inserts the linebreak. The Print Formatted allows us to accept certain types into our print statements with %s
. Basically Go inserts the string (%s
) that we pass in after the comma (something
) and substitutes it, the output should remain:
Hello World!
If you're every unsure what type a variable is, you can try %v
to just output it no matter if it's a string
(text), a %d
(decimal) or something else.
Loops in Go
To write loops in go, you can use a familiar for loop:
func main() {
i := 1
for i <= 3 {
fmt.Println(i)
i = i + 1
}
}
More Go Examples
If you want to check out more Go examples, head over to gobyexample.com, because they have a ton of great examples if you want to explore how familiar go already is.