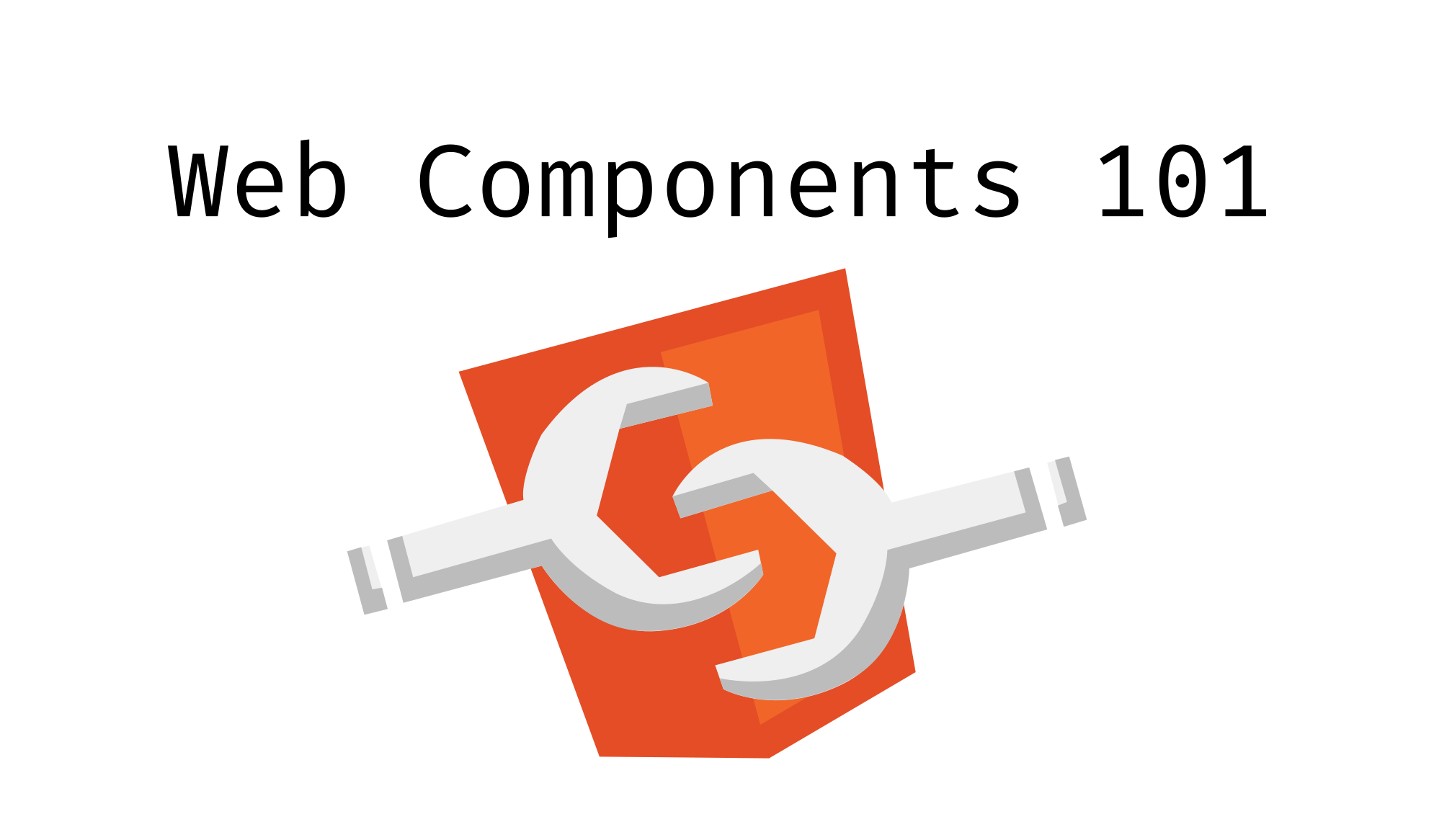
Web Components 101: Hello World
Web Components are a way to encapsulate functionality into little self-contained parts that can be reused and adapted like you're used to from frontend libraries like React, Vue, Angular or Ember. The special thing about Web components is that they work straight in your browser.
When it comes to support, a lot of features are supported in all major browsers, as can bee checked out at caniuse.com.
As you might have seen, web components usually are understood as several sub-parts, mostly:
- HTML Templates (the
<template>
tag) - Custom Elements (we'll create one below)
- Shadow DOM (the new, cooler iframe that isolates stuff like video players and other comprehensive components a little)
- ES Modules (as in ECMA Script, basically modularisation or better JavaScript Classes)
Let's go ahead and create the easiest possible web component that we can possibly think of, the <hello-world>
. Now HTML tags are kind of a free for all kind of buffet, except that you not only can use which ever you want, you can also create which ever you like. So we'll just name ours what ever the hell we want, while observing the safety restrictions like no numbers or special characters or other shenanigans.
If you want to jump straight to the interactive demo, scroll down!
Right, let's dive in, first we need a super boring HTML page:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Hello World</title>
<style>
article {
max-width: 38rem;
padding: 2rem;
margin: auto;
font-family: "Gill Sans", "Gill Sans MT", Calibri, "Trebuchet MS",
sans-serif;
}
</style>
</head>
<body>
<article>
<h1>Hello World of Web Components</h1>
<p>This is a web components demo! Now let's go:</p>
</article>
</body>
</html>
Do you see any web components? Me neither. Also I stole these 58 bytes of CSS. Let's add one! For starters, I'll add this below the first <p>
tag.
<!-- DANGER -->
<!-- WEB COMPONENTS -->
<!-- BEYOND THIS POINT -->
<hello-world></hello-world>
The First Custom Element
Next, we're going to add a <script>
tag after the <article>
with the real juicy stuff, our custom element:
// ES6 class
class HelloWorld extends HTMLParagraphElement {
// constructor is called when the element is displayed
constructor() {
super();
// create a shadow dom
const shadow = this.attachShadow({ mode: "closed" });
// create a span element
const text = document.createElement("span");
// set the content to 'Hello World'
text.textContent = "Hello World";
// insert our created element into our shadow DOM, causing it to appear
shadow.appendChild(text);
}
}
// make sure that the <hello-world></hello-world>
// or simply <hello-world /> is recognised as this element
customElements.define("hello-world", HelloWorld, { extends: "p" });
Alright, I know that's a lot of code to make a "Hello World" appear anywhere. It's not super useful to us right now, but this shows us a couple of things and is an important stepping stone to the next level!
Let's break down what's going on in this example:
- our JavaScript
class
extends the existingHTMLParagraphElement
, see more possible extendable classes - our
shadow
variable is our little document within a document and we don't need a or similar to render to - the
shadow.appendChild
makes sure that anything at all gets rendered customElements.define
makes sure that the browser gets the connection between our classHelloWorld
and the element tag
Interactive fiddle:
Great! You've created your first custom element, if you've coded along of course! Now what's the point, you might ask? Well you can now create rich elements and interfaces without relying on the usual suspects of frameworks or just apply this when you have a task where a framework would be overkill.
Further Reading
If you've gotten curious about Web Components and the future of the web that might no longer have any framework wars going on, check out these links:
Last, but not least: Shout out to the friendly people at ideanote.io for hosting a study group in their office this week to bring togehter web component curious people!